기본 골자
public class lamda01 {
public static void main(String[] args) {
Sample01Function f = () -> {
System.out.println("샘플01테스트 출력1");
System.out.println("샘플01테스트 출력2");
};
f.test();
Sample02Function f2 = (a) -> a;
System.out.println(f2.test("샘플02테스트 출력1"));
Sample02Function f3 = a -> { // 괄호를 붙이면 반환값을 줘야함
return a;
};
System.out.println(f3.test("샘플02테스트 출력2"));
}
}
@FunctionalInterface
interface Sample01Function {
public void test();
}
@FunctionalInterface
interface Sample02Function {
public String test(String a);
}
결과
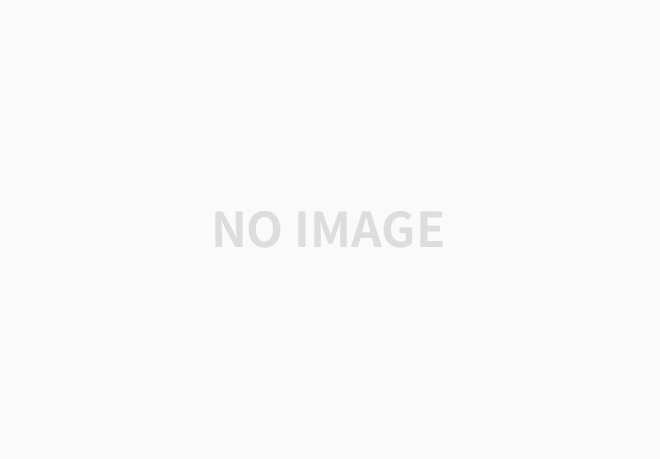
내장 함수 이용
public class lamda02 {
public static void main(String[] args) {
Function<Integer, String> r = t -> {
String result = "";
for(int i = 0 ; i < t; i++) {
result += "test\n";
}
return result;
};
System.out.println(r.apply(5));
}
}
public class lamda03 {
public static void main(String[] args) {
// Runnable
Runnable r = () -> {
System.out.println("Runnable은 매개변수는 없고 반환 자료형도 없다.");
};
r.run();
// Supplier
//Supplier<String> s = () -> "";
Supplier<String> s = () -> {
return "Supplier는 매개변수는 없고 반환 자료형은 있다.";
};
System.out.println(s.get());
MessageCenter mc = new MessageCenter("Hello! Lamda!");
Supplier<MessageCenter> param = () -> mc;
MessageCenter main = getMsg(param);
System.out.println(main);
}
public static MessageCenter getMsg(Supplier<MessageCenter> mc) {
return mc.get();
}
}
class MessageCenter {
private final String msg;
MessageCenter(String msg) {
this.msg = msg;
}
public String toString() {
return msg;
}
}
결과
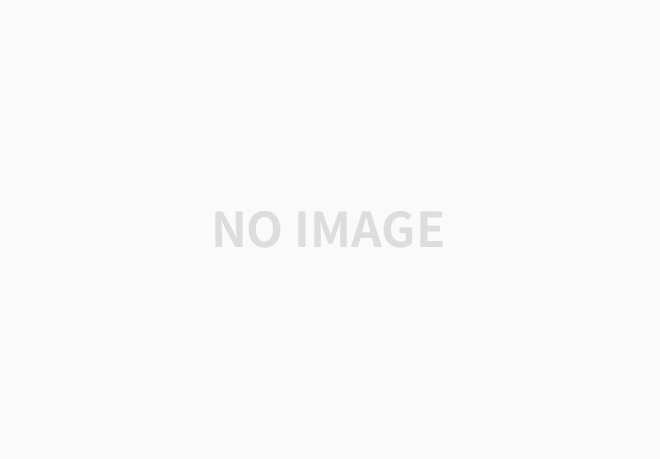
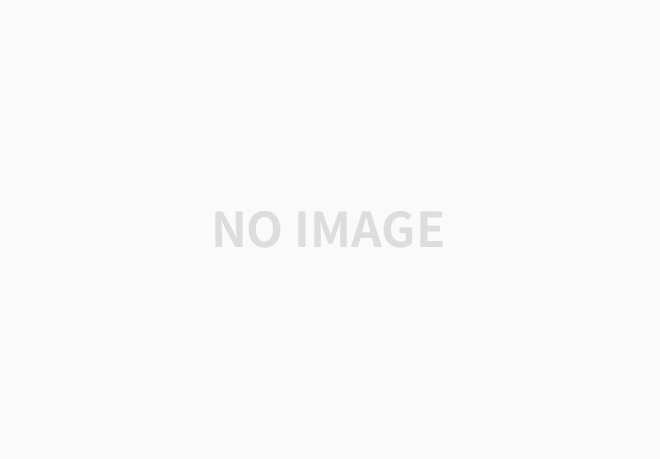
함수형 인터페이스 API
인터페이스명 | 반환 자료형 | 메서드 | 매개변수 |
Ruunnable | void | run() | 없음 |
Supplier | T | get() | 없음 |
Consumer | void | accept(T t) | 1개 |
Function<T,R> | R | apply(T t) | 1개 |
Predicate | boolean | test(T t) | 1개 |
UnaryOperator | T | apply(T t) | 1개 |
BiConsumer<T,U> | void | accept(T t, U u) | 2개 |
BiFunction<T,U,R> | R | apply(T t, U u) | 2개 |
BiPredicate<T,U> | boolean | test(T t, U u) | 2개 |
BinaryOperator | T | apply(T t, U u) | 2개 |
'Dev > JAVA' 카테고리의 다른 글
제네릭(Generic) 이란 ? (0) | 2023.11.17 |
---|